R3vo/Sandbox – User
Jump to navigation
Jump to search
↑ Back to spoiler's top
↑ Back to spoiler's top
= Arma 3: Module Configuration
This page can be considered a hub when it comes to Modules. Here you will find everything you need to know when it comes to creating modules, available modules in Arma 3 and existing module documentation.
General Information
- Modules are executed before initServer.sqf, initPlayerLocal.sqf and initPlayerServer.sqf (See Initialisation Order)
- Modules are very easy to set up, even for unexperienced users
- They can easily be used to create mission templates
Create a Module Addon
- Make a folder named TAG_addonName a create a config.cpp file in it.
- Inside, declare a CfgPatches class with your addon and its modules (in the units array). Without this, the game would not recognise the addon.
- Make sure the addon and all objects start with your tag, e.g. TAG_.
Example
class CfgPatches { class TAG_addonName { units[] = { "TAG_ModuleNuke" }; requiredVersion = 1.0; requiredAddons[] = { "A3_Modules_F" }; }; };
Module Category
- Modules are placed into basic categories which makes finding a desired module easier.
You can use on of the existing categories (See table to the right) or create your own category (see example below).
class | displayName |
---|---|
Effects | Effects |
Events | Events |
Modes | Gameplay Modes |
GroupModifiers | Group Modifiers |
Intel | Intel |
NO_CATEGORY | Misc |
Multiplayer | Multiplayer |
ObjectModifiers | Object Modifiers |
Sites | Sites |
StrategicMap | Strategic |
Supports | Supports |
Example
class CfgFactionClasses { class NO_CATEGORY; class TAG_explosions: NO_CATEGORY { displayName = "Explosions"; }; };
Creating the Module Config
- All in-game objects (soldiers, vehicles, buildings, logics, modules, ...) are defined in CfgVehicles class.
- All modules must inherit from Module_F parent class, either directly or through some additional sub-parent.
- Modules functions are by default not executed when in Eden Editor workspace. It can be enabled using is3DEN property, but that will also change format of function params.
Example:
class CfgVehicles
{
class Logic;
class Module_F : Logic
{
class AttributesBase
{
class Default;
class Edit; // Default edit box (i.e. text input field)
class Combo; // Default combo box (i.e. drop-down menu)
class Checkbox; // Default checkbox (returned value is Boolean)
class CheckboxNumber; // Default checkbox (returned value is Number)
class ModuleDescription; // Module description
class Units; // Selection of units on which the module is applied
};
// Description base classes (for more information see below):
class ModuleDescription
{
class AnyBrain;
};
};
class TAG_Module_Nuke : Module_F
{
// Standard object definitions:
scope = 2; // Editor visibility; 2 will show it in the menu, 1 will hide it.
displayName = "Nuclear Explosion"; // Name displayed in the menu
icon = "\TAG_addonName\data\icon_Nuke_ca.paa"; // Map icon. Delete this entry to use the default icon.
category = "Effects";
function = "TAG_fnc_moduleNuke"; // Name of function triggered once conditions are met
functionPriority = 1; // Execution priority, modules with lower number are executed first. 0 is used when the attribute is undefined
isGlobal = 1; // 0 for server only execution, 1 for global execution, 2 for persistent global execution
isTriggerActivated = 1; // 1 for module waiting until all synced triggers are activated
isDisposable = 1; // 1 if modules is to be disabled once it is activated (i.e. repeated trigger activation will not work)
is3DEN = 1; // 1 to run init function in Eden Editor as well
curatorCanAttach = 1; // 1 to allow Zeus to attach the module to an entity
curatorInfoType = "RscDisplayAttributeModuleNuke"; // Menu displayed when the module is placed or double-clicked on by Zeus
// 3DEN Attributes Menu Options
canSetArea = 1; // Allows for setting the area values in the Attributes menu in 3DEN
canSetAreaShape = 1; // Allows for setting "Rectangle" or "Ellipse" in Attributes menu in 3DEN
class AttributeValues
{
// This section allows you to set the default values for the attributes menu in 3DEN
size3[] = { 100, 100, -1 }; // 3D size (x-axis radius, y-axis radius, z-axis radius)
isRectangle = 0; // Sets if the default shape should be a rectangle or ellipse
};
// Module attributes (uses https://community.bistudio.com/wiki/Eden_Editor:_Configuring_Attributes#Entity_Specific):
class Attributes : AttributesBase
{
// Arguments shared by specific module type (have to be mentioned in order to be present):
class Units : Units
{
property = "TAG_Module_Nuke_Units";
};
// Module-specific arguments:
class Yield : Combo
{
property = "TAG_Module_Nuke_Yield"; // Unique property (use "<tag>_<moduleClass>_<attributeClass>" format to ensure that the name is unique)
displayName = "Nuclear weapon yield"; // Argument label
tooltip = "How strong will the explosion be"; // Tooltip description
typeName = "NUMBER"; // Value type, can be "NUMBER", "STRING" or "BOOL"
defaultValue = "50"; // Default attribute value. Warning: this is an expression, and its returned value will be used (50 in this case).
// Listbox items
class Values
{
class 50Mt { name = "50 megatons"; value = 50; };
class 100Mt { name = "100 megatons"; value = 100; };
};
};
class Name : Edit
{
displayName = "Name";
tooltip = "Name of the nuclear device";
property = "TAG_Module_Nuke_Name";
// Default text for the input box:
defaultValue = """Tsar Bomba"""; // Because this is an expression, one must have a string within a string to return a string
};
class ModuleDescription : ModuleDescription {}; // Module description should be shown last
};
// Module description (must inherit from base class, otherwise pre-defined entities won't be available)
class ModuleDescription : ModuleDescription
{
description = "Short module description"; // Short description, will be formatted as structured text
sync[] = { "LocationArea_F" }; // Array of synced entities (can contain base classes)
class LocationArea_F
{
description[] = { // Multi-line descriptions are supported
"First line",
"Second line"
};
position = 1; // Position is taken into effect
direction = 1; // Direction is taken into effect
optional = 1; // Synced entity is optional
duplicate = 1; // Multiple entities of this type can be synced
synced[] = { "BluforUnit", "AnyBrain" }; // Pre-defined entities like "AnyBrain" can be used (see the table below)
};
class BluforUnit
{
description = "Short description";
displayName = "Any BLUFOR unit"; // Custom name
icon = "iconMan"; // Custom icon (can be file path or CfgVehicleIcons entry)
side = 1; // Custom side (determines icon color)
};
};
};
};
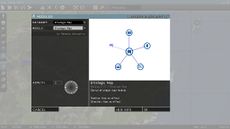
2D Editor: The description is available after clicking on "Show Info" button when editing the module
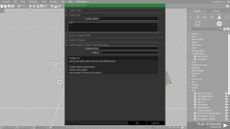
Eden Editor: The description is available after opening the modules' attributes window
Pre-defined sync preview entities can be:
Class | Descripton |
---|---|
Anything | Any object - persons, vehicles, static objects, etc. |
AnyPerson | Any person. Not vehicles or static objects. |
AnyVehicle | Any vehicle. No persons or static objects. |
GroupModifiers | Group Modifiers |
AnyStaticObject | Any static object. Not persons or vehicles. |
AnyBrain | Any AI or player. Not empty objects |
AnyAI | Any AI unit. Not players or empty objects |
AnyPlayer | Any player. Not AI units or empty objects |
EmptyDetector | Any trigger |
Configuring the Module Function
- Place class CfgFunctions to config.cpp. See Arma 3: Functions Library for more info about functions configuration.
Example
class CfgFunctions { class TAG { class Effects { file = "\TAG_addonName\functions"; class moduleNuke {}; }; }; };
Writing the Module Function
- Create the functions folder within the addon folder and place *.sqf or *.fsm files there.
- Example: \TAG_addonName\functions\fn_moduleNuke.sqf
- Input parameters differ based on value of is3DEN property.
Default Example (is3DEN = 0)
params [
["_logic", objNull, [objNull]], // Argument 0 is module logic
["_units ", [], [[]]], // Argument 1 is a list of affected units (affected by value selected in the 'class Units' argument))
["_activated", true, [true]] // True when the module was activated, false when it is deactivated (i.e., synced triggers are no longer active)
];
// Module specific behavior. Function can extract arguments from logic and use them.
if (_activated) then
{
// Attribute values are saved in module's object space under their class names
private _bombYield = _logic getVariable ["Yield", -1]; // (as per the previous example, but you can define your own)
hint format ["Bomb yield is: %1", _bombYield]; // will display the bomb yield, once the game is started
};
// Module function is executed by spawn command, so returned value is not necessary, but it is good practice.
true;
Example Eden Editor (is3DEN = 1)
When is3DEN = 1 is set in module config, different, more detailed params are passed to the function.
params [
["_mode", "", [""]],
["_input", [], [[]]]
];
switch _mode do
{
// Default object init
case "init":
{
_input params [
["_logic", objNull, [objNull]], // Module logic
["_isActivated", true, [true]], // True when the module was activated, false when it is deactivated
["_isCuratorPlaced", false, [true]] // True if the module was placed by Zeus
];
// ... code here...
};
// When some attributes were changed (including position and rotation)
case "attributesChanged3DEN": {
_input params [
["_logic", objNull, [objNull]]
];
// ... code here...
};
// When added to the world (e.g., after undoing and redoing creation)
case "registeredToWorld3DEN": {
_input params [
["_logic", objNull, [objNull]]
];
// ... code here...
};
// When removed from the world (i.e., by deletion or undoing creation)
case "unregisteredFromWorld3DEN": {
_input params [
["_logic", objNull, [objNull]]
];
// ... code here...
};
// When connection to object changes (i.e., new one is added or existing one removed)
case "connectionChanged3DEN": {
_input params [
["_logic", objNull, [objNull]]
];
// ... code here...
};
// When object is being dragged
case "dragged3DEN": {
_input params [
["_logic", objNull, [objNull]]
];
// ...code here...
};
};
true;